Routing rulesets
Email routing rules for controlling which emails can be sent or received from inboxes.
Quick links:
About rulesets
Inbox rulesets can be used to create rules about which emails can be sent or received from an inbox. Rulesets are applied to inboxes or at the account level and can be used to control which emails are allowed to be sent or received from inboxes or accounts.
Find rulesets in dashboard
You can create and manage inbox rulesets in the MailSlurp dashboard or using API integrations.
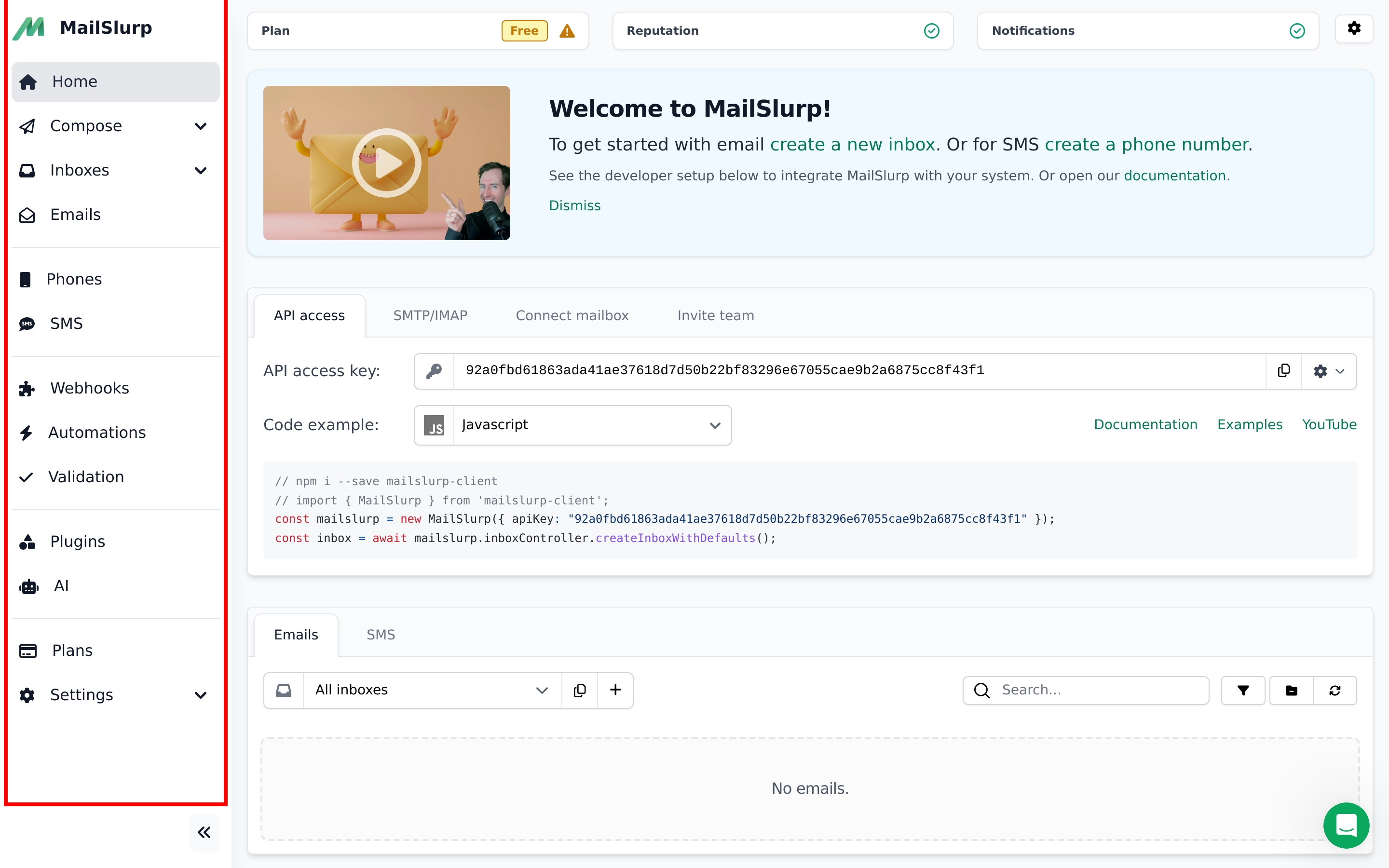
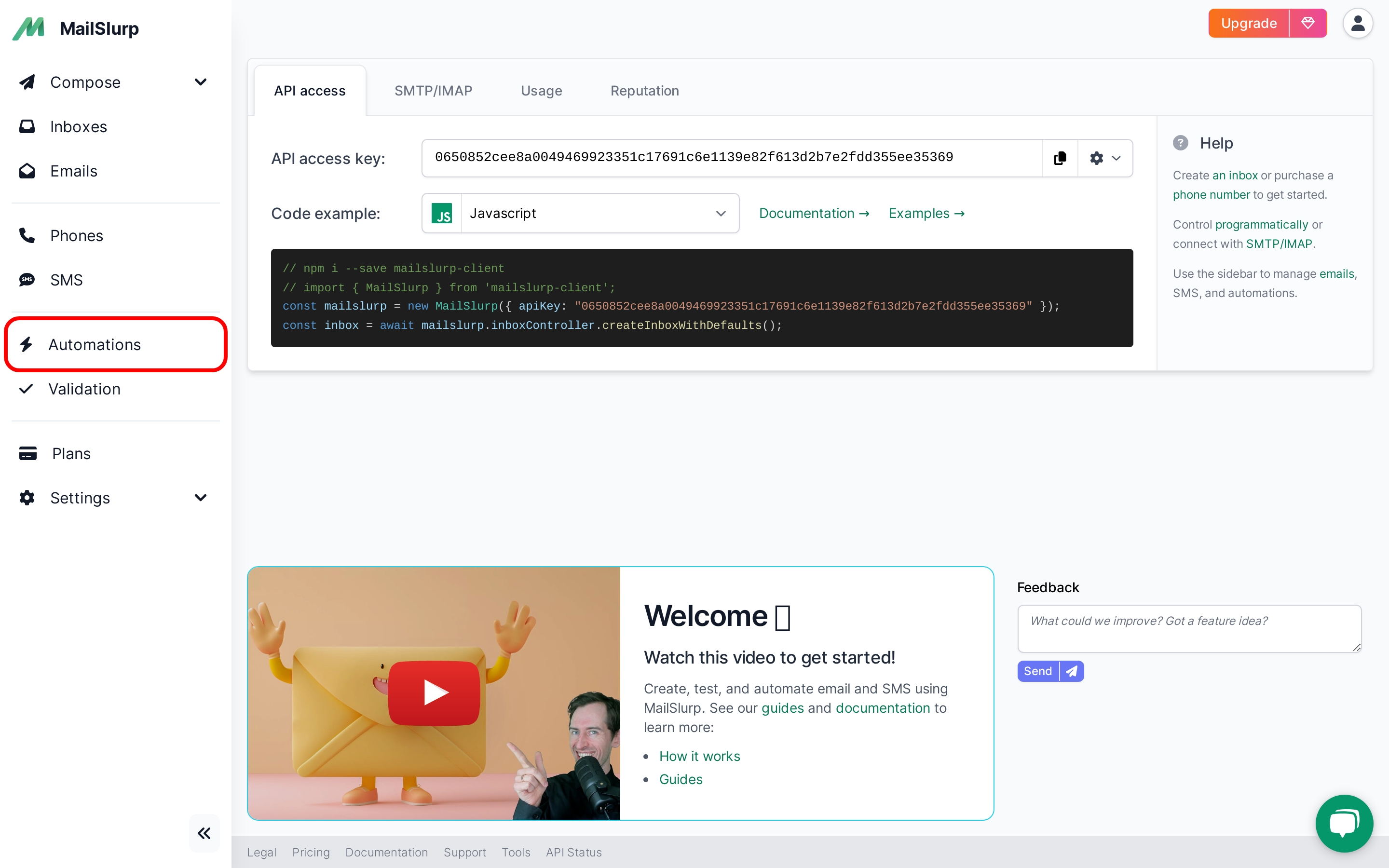
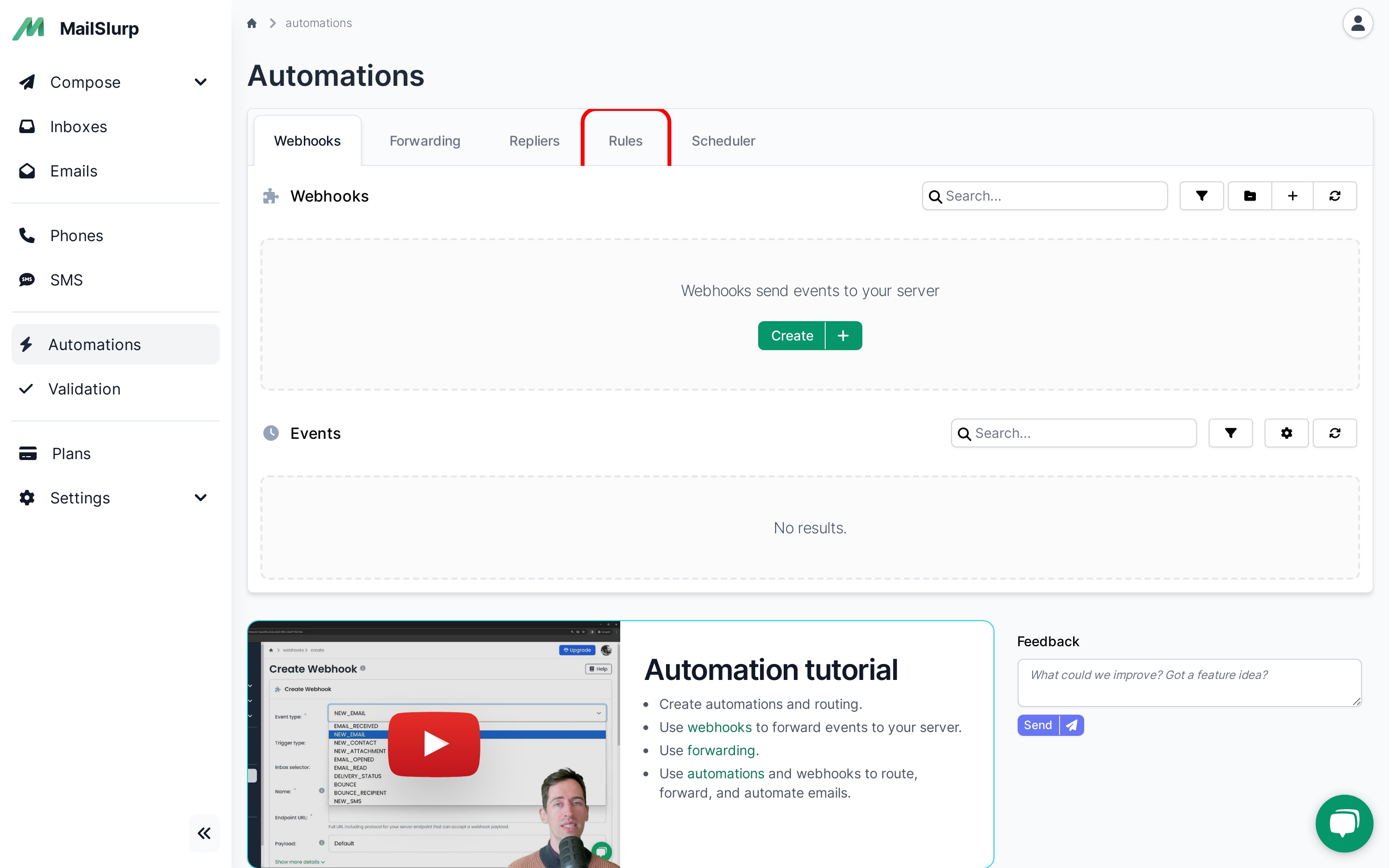
Creating rulesets
Rulesets can apply to a specific inbox or be account wide. They can also apply to inbound or outbound emails. You can create rulesets in code like so:
const ruleset = await mailslurp.inboxRulesetController.createNewInboxRuleset({
createInboxRulesetOptions: {
action: CreateInboxRulesetOptionsActionEnum.BLOCK,
scope: CreateInboxRulesetOptionsScopeEnum.SENDING_EMAILS,
target: '*',
},
inboxId: undefined,
});
In the dashboard you can create rulesets by clicking the Create ruleset
button on the ruleset page.
Testing rules
You can test whether email addresses can send to or receive from an inbox using the ruleset tester. This is useful for debugging rulesets. It can be found on the ruleset index page:
Rule precedence
Rules apply incrementally and favour ALLOW
over BLOCK
. For instance if you have a global BLOCK
rule for all email addresses and an ALLOW
for a particular domain, any emails from that domain will be permitted. Here is an example of combined rules and how to test them. First we create two rulesets in code:
const inbox = await mailslurp.createInbox();
// block all inbound emails
await mailslurp.inboxRulesetController.createNewInboxRuleset({
createInboxRulesetOptions: {
action: CreateInboxRulesetOptionsActionEnum.BLOCK,
scope: CreateInboxRulesetOptionsScopeEnum.RECEIVING_EMAILS,
target: '*',
},
inboxId: inbox.id
});
// allow from a single domain
await mailslurp.inboxRulesetController.createNewInboxRuleset({
createInboxRulesetOptions: {
action: CreateInboxRulesetOptionsActionEnum.ALLOW,
scope: CreateInboxRulesetOptionsScopeEnum.RECEIVING_EMAILS,
target: '*@mydomain.com',
},
inboxId: inbox.id
});
Next we verify that certain domains can send to the inbox:
// test receiving from allowed domain
const test1 = await mailslurp.inboxRulesetController.testInboxRulesetReceiving({
testInboxRulesetReceivingOptions: {
inboxId: inbox.id,
fromSender: 'hello@mydomain.com'
}
})
expect(test1.canReceive).toEqual(true)
// test receiving from any other domain is blocked
const test2 = await mailslurp.inboxRulesetController.testInboxRulesetReceiving({
testInboxRulesetReceivingOptions: {
inboxId: inbox.id,
fromSender: 'hi@other-domain.com'
}
})
expect(test2.canReceive).toEqual(false)
What happens to blocked emails
Emails that are received but blocked by a rule and saved in the MissedEmails
section of the app and can also be accessed via webhooks and the API.
Forwarding and replies
If you wish to match recipients and automatically reply or forward see those features: