Forwarding
Inbox forwarding allows you to create rules that match inbound emails and forward them automatically to other email addresses.
Quick links
Find forwarders in dashboard
You can create and manage inbox forwarders in the MailSlurp dashboard or using the API.
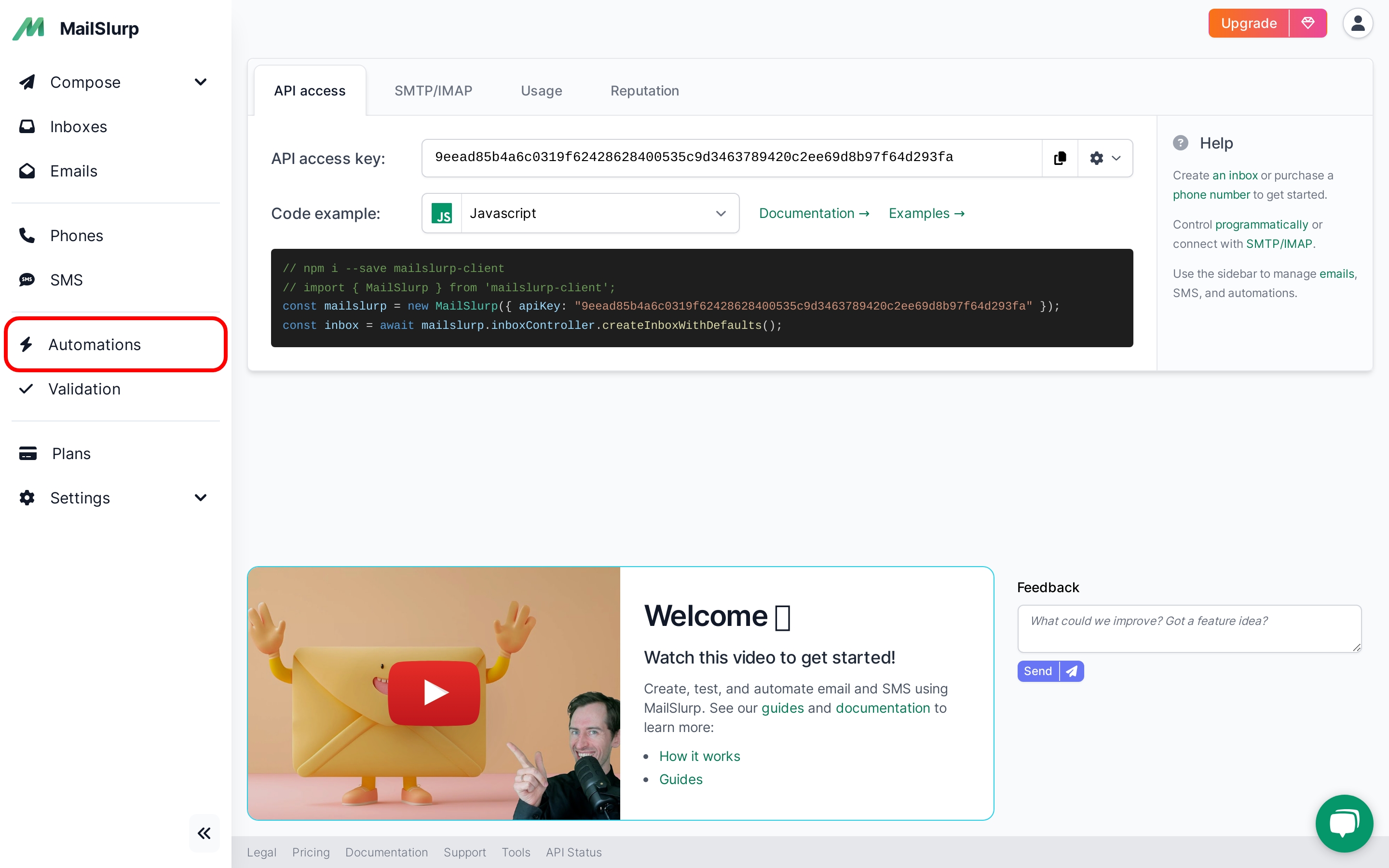
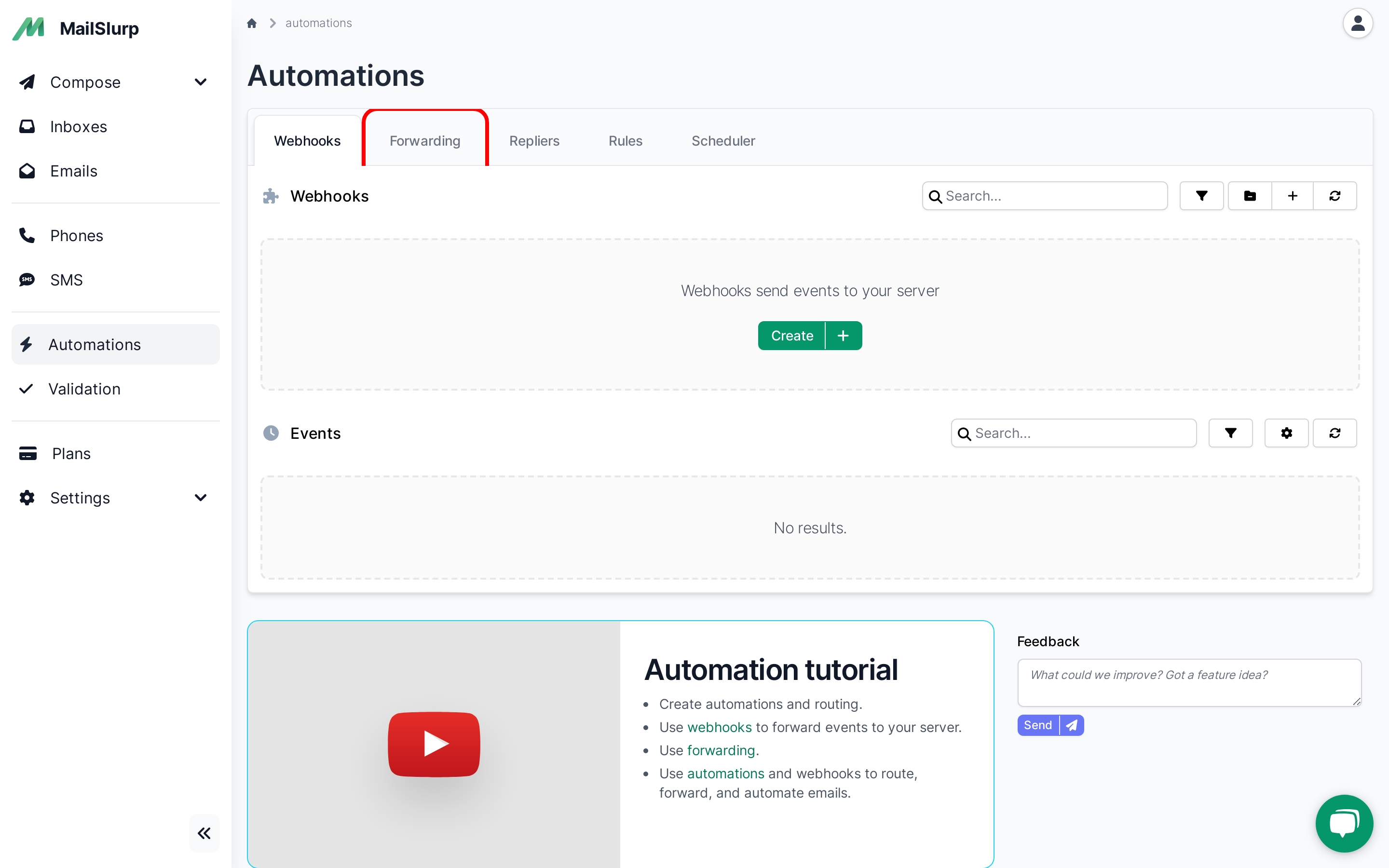
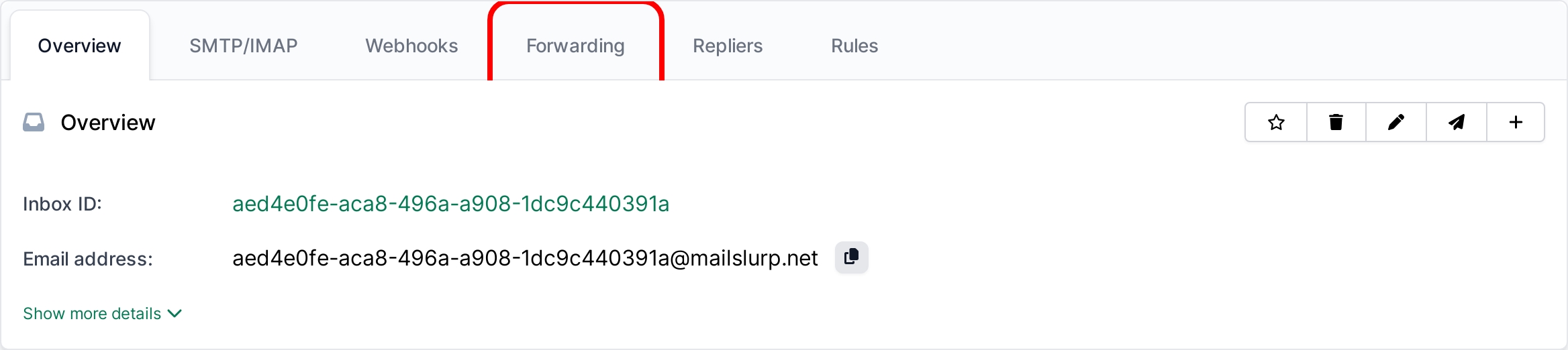
How forwarding works
Inbox forwarding rules can be attached to inboxes and catch-all inboxes and are applied to inbound emails. Rules can match on email fields such as subject, or sender, and can be used to forward emails to other email addresses.
When an inbox with a forwarding rule attached receives an inbound email the rules are evaluated. For each matching rule the email is forwarded to the attached rule recipient. Both the original inbox and the recipient inbox receive the inbound email.
Creating inbox forwarders
You can create inbox forwarders in the dashboard or using the API.
Matching inbound emails
You can create rules to match on a variety of email properties:
const forwarderFields = [
CreateInboxForwarderOptionsFieldEnum.SENDER,
CreateInboxForwarderOptionsFieldEnum.RECIPIENTS,
CreateInboxForwarderOptionsFieldEnum.ATTACHMENTS,
CreateInboxForwarderOptionsFieldEnum.SUBJECT
]
To create an inbox forward rule in code we can do so like this:
await mailslurp.inboxForwarderController.createNewInboxForwarder({
inboxId: inbox2.id,
createInboxForwarderOptions: {
field: CreateInboxForwarderOptionsFieldEnum.SENDER,
match: inbox1.emailAddress!,
forwardToRecipients: [inbox3.emailAddress!],
},
});
Pattern match wildcards
You can use an *
asterisk to match all characters after a given pattern. Here is an example of matching subjects using this method.
await mailslurp.inboxForwarderController.createNewInboxForwarder({
inboxId: inbox1.id,
createInboxForwarderOptions: {
field: CreateInboxForwarderOptionsFieldEnum.SUBJECT,
match: 'Invoice AB-*',
forwardToRecipients: [inbox2.emailAddress!],
},
});
We can test this pattern match using MailSlurp inboxes. Here we email the above inbox and expect the forwarding rule to match.
const sent = await mailslurp.inboxController.sendEmailAndConfirm({
inboxId: inbox3.id!!,
sendEmailOptions: {
to: [inbox1.emailAddress!!],
subject: 'Invoice AB-123',
},
});
Using the waitFor methods we can wait for the forwarded email to arrive in forwarding recipient inbox.
// see that inbox1 gets the original email
const receivedEmail = await mailslurp.waitController.waitForLatestEmail({
inboxId: inbox1.id,
timeout: 60000,
unreadOnly: true,
});
expect(receivedEmail.subject).toContain('Invoice AB-123');
// see that inbox2 also gets a forwarded email
const forwardedEmail = await mailslurp.waitForLatestEmail(
inbox2.id,
60000,
true
);
expect(forwardedEmail.subject).toContain('Invoice AB-123');
Tracking forwarding events
Each forwarding event is recorded and can be accessed via the API or dashboard. You can use this to track the number of emails forwarded by a given rule or inbox.
Advanced examples
Here is an example of forwarding between inboxes.
const mailslurp = new MailSlurp({ apiKey: process.env.apiKey });
// create two inboxes for testing
const inbox1 = await mailslurp.inboxController.createInboxWithDefaults();
const inbox2 = await mailslurp.inboxController.createInboxWithDefaults();
const inbox3 = await mailslurp.inboxController.createInboxWithDefaults();
// add auto forwarding rule to inbox 2
await mailslurp.inboxForwarderController.createNewInboxForwarder({
// attach rule to inbox 2
inboxId: inbox2.id,
createInboxForwarderOptions: {
// filter emails that match the sender from inbox 1 and send to inbox 3
field: CreateInboxForwarderOptionsFieldEnum.SENDER,
match: inbox1.emailAddress,
forwardToRecipients: [inbox3.emailAddress],
},
});
// send email from inbox1 to inbox2
await mailslurp.sendEmail(inbox1.id!!, {
to: [inbox2.emailAddress!!],
subject: 'Hello',
});
// see that inbox2 gets the original email
const receivedEmail = await mailslurp.waitForLatestEmail(
inbox2.id,
60000,
true
);
expect(receivedEmail.subject).toContain('Hello');
// see that inbox3 gets a forwarded email
const forwardedEmail = await mailslurp.waitForLatestEmail(
inbox3.id,
60000,
true
);
expect(forwardedEmail.subject).toContain('Hello');
// check the sent messages for inbox2 to find the forwarded message
const sentFromInbox2 = await mailslurp.sentController.getSentEmails({
inboxId: inbox2.id,
});
expect(sentFromInbox2.totalElements).toEqual(1);
const forwardedMessage = sentFromInbox2.content[0];
expect(forwardedMessage.to).toEqual([inbox3.emailAddress]);
expect(forwardedMessage.from).toEqual(inbox2.emailAddress);
Other resources
You may also be interested in webhooks, rulesets, and auto-replies.